The Perils of Visual Basic
# 2023-09-29
# The many footguns of VB.Net.
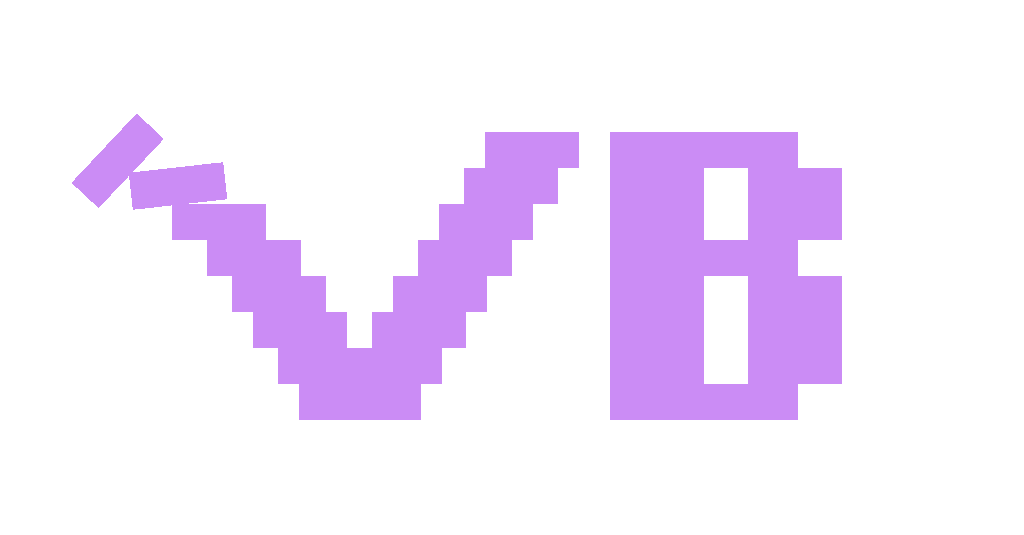
Visual Basic was designed by Microsoft be a user-friendly yet powerful language for building desktop applications. While VB.NET is a much less hated language than the classic Visual Basic 6.0 or VBA, it still hangs on to a lot of the baggage and poor design decisions of the past. Most developers would put the more active and feature-rich C# as their preferred language on the .NET platform, but often you won't have a choice. Visual Basic is still a language you can be very productive with, but there are hidden trip-wires everywhere.
First off, we'll start with an obvious syntax difference that might catch you out if coming from any other modern programming language. When first learning to program, getting used to interpreting the equality sign =
as assignment, rather than its mathematical meaning, usually stumbles beginners. Visual Basic takes this one step further by scrapping the double equality (==
) entirely and instead uses the same symbol for equivalence too. This means one symbol can have two different meanings depending on where it's used. This can quickly create confusing code for people unfamiliar with Visual Basic.
Dim n As Integer
n = 0 ' Assignment
If n = 1 Or n = 2 Then ' Equality checking
n = 2 ' Assignment
End If
If muscle memory takes over and you happen to make your operator two characters long, Visual Studio can send you on a hell of a goose chase with a beautifully vague "Expression expected" error message, since in Visual Basic's eyes, your equality operator is performing on an expression and another equality operator.
Weak Typing by Default
Obviously, weak typing is a weird one. This means a value is allowed to vary from what it's type indicates it should be. These looser typing rules may give you some seriously unexpected results with the language performing implicit type conversions at runtime where it sees fit. It means you can cut corners, be lazy and work faster.
The classic example is C's weak typing system due to its pointer arithmetic. It exposes a pointer type as if it was an integer, allowing the programmer to bounce across memory. JavaScript is another example that has a much more prevalent weak typing system.
When we do something like the code below, Visual Basic decides that it makes sense to perform the implicit conversion of "20"
into 20
in order to perform the addition to get 30
. Although +
is primarily used as an addition operator, you could easily argue that the result could be "2010"
.
Dim x = "20" + 10 ' 30
Believe it or not, the resulting 30
is a double. The compiler performs a double conversion behind the scenes without being explicitly told to do so. Why double? It's probably just a better catch-all.
Dim x = Convert.ToDouble("20") + 10
Dim x = 20 + 10 ' 30 (Integer)
Dim z = "20" + 10 ' 30 (Double)
And if we declare x
as a string, it would convert the resulting 30
into "30"
.
Dim x As String = "20" + 10 ' "30"
Dim y As String = 30 ' A completely valid "30"
Weak typing also means that you can assign an integer variable with a double value, and not only will give no compiler errors or warnings (since it is perfectly valid), but it will implicitly round to the nearest integer(!). This is just a fantastic source of bugs. It's almost like they didn't want us to succeed.
Dim d1 As Double = 5.12
Dim i1 As Integer = d1
Dim i2 As Integer = 5.64
Dim i3 As Integer = 1/3
Console.WriteLine(d1) ' 5.12
Console.WriteLine(i1) ' 5
Console.WriteLine(i2) ' 6
Console.WriteLine(i3) ' 0
Unbelievably, this isn't even a particularly harsh example. If you accidentally attempt to use a string as a date, instead of any kind of warning... it will just... have a go??
Sub DoSomething(myDate As Date)
Console.WriteLine(myDate) ' 2/1/2009 3:03:00AM
Console.WriteLine(myDate.Day) ' 1
Console.WriteLine(myDate.Month) ' 2
Console.WriteLine(myDate.Year) ' 2009
End Sub
Sub Main()
Dim vaguelyADate As String = "2. 1-9 3:3"
DoSomething(vaguelyADate)
End Sub
The weak typing system of VB.NET can so easily catch you out, especially since C# is strongly-typed. There is a way to disable weak typing, but unless you are starting a new project, turning it off will probably make your screen light up like a Christmas tree.
' Enable strong typing
Option Strict On
Case Insensitivity
Where some languages reserve title case for classes or uppercase for constants to make it quicker to digest the semantics, Microsoft went a different route and made Visual Basic case insensitive. You could write your entire VB.NET application in caps if you wanted. Whilst this means you can relax with the shift key, this opens up new doors to create code that is difficult to read, especially coming from languages where Name
and name
are two different things entirely.
dim uSErnAmEFOrlogIN as string
console.writeline(USERNAMEFORLOGIN) ' Valid, although probably corrected by an IDE
Optional Parenthesis
Visual Basic makes code readability harder still by making parenthesis after a method completely optional, making it harder to distinguish between a property or a method. It also uses parenthesis for both method calls and array accesses. Fun!
Function GetArray() As Integer()
Return New Integer() {1, 2, 4, 8}
End Function
Function GetArray(i As Integer) As Integer()
Return New Integer() {16, 32, 64, 128}
End Function
Sub Main()
String.Join(",", GetArray) ' 1,2,4,8
String.Join(",", GetArray(2)) ' 16,32,64,128
String.Join(",", GetArray()) ' 1,2,4,8
GetArray(2)(2) ' 64
GetArray()(2) ' 4
End Sub
Concatenation
Concatenation of strings in Visual Basic is achieved with a concatenation operator, denoted by an ambersand &
.
Dim str1 As String = "hello"
Dim str2 As String = "world"
Dim str3 As String = str1 & " " & str2
However, the addition operator +
also works, giving two ways to achieve the same thing!
While the &
operator was indented to concatenate strings together, the +
operator is primarily used for adding numbers, but it can also concatenate strings. Therefore if a number gets involved in your concatenation, paired with VB's dynamic typing, things can go unexpectedly south.
"abc" & "def" ' "abcdef"
"abc" + "def" ' "abcdef"
"123" & "456" ' "123456"
"123" + "456" ' "123456"
"123" & 456 ' "123456"
"123" + 456 ' 579
"abc" & 456 ' "abc456"
"abc" + 456 ' System.InvalidCastException: Conversion from string "abc" to type 'Double' is not valid.
Depending on whether your string is numeric, or whether you concatenate with &
or +
, your could end up with wildly different results or even crash your program.
For Loop
In most languages, the exit condition for a for loop is reevaluated with each iteration, so it is constantly up-to-date.
As expected, the JavaScript code below will never terminate, as new values are added to the numbers list, changing the value of numbers.length
.
let numbers = [0, 0, 0]
for (let i = 0; i < numbers.length; i++) {
numbers.push(1)
console.log(i)
}
In Visual Basic, the exit condition is evaluated once and only once at the start of the for loop.
The code below will print the numbers 0, 1, 2 only.
Dim numbers As New List(Of Integer)({0, 0, 0})
For i As Integer = 0 To numbers.Count - 1
numbers.Add(0)
Console.WriteLine(i)
Next
There are scenarios where this is beneficial from a performance point of view, but this unusual behaviour can be an easy source of bugs.
Inconsistent Syntax
Now this is only a minor inconvenience, but if you only occassionally program in Visual Basic, remembering syntax can be a struggle, especially when it comes to loops.
If you're writing a For
loop, you just need to remember to close the section with Next
, or Next i
where i
is your looping variable if you want to. And the same with For Each
. That's fair enough.
But with a While
loop, now we need an End While
? Surely Next
would have been fine (although this is still better than Wend
in VBA).
And a Do
loop? We need to finish with a Loop While
or Loop Until
?
And then there's Do While
and Do Until
loops. These need to close with a Loop
.
That makes nine different ways to create a loop. I understand this makes it more idiomatic for beginners, but this is just excessive and it only loads you with more to remember. Fortunately, IDEs like Visual Studio will automatically create the closing tag for you by default.
Baggage
Visual Basic has very old roots, and the code you are looking at is probably legacy. It's partly due to this that many of the decades-old conventions still live on even when they no longer bring any real benefit. It's still very common to find code written recently that subscribes to the use of Hungarian Notation, that is, variable names prefixed with their type, probably to remain consistent with the wider codebase. age
becomes intAge
and name
becomes strName
. It was previously used to express the intended type of a variable before true type definitions existed. These days we have a proper type system and intelligent IDEs. The problem now is that the moment you decide to move away from this convention you will be working with two different naming conventions (assuming your codebase is not small).
It was also common to declare all the variables needed at the top of a subroutine or function, with the intention of setting their values and using them further down. Pair this with the fact that variables are automatically assigned a default zero-value (that may be a valid value within the context of the scope), and we have a recipe for a lot of sneaky bugs. If a function ever gets quite long (and it will), this problem only gets much harder to deal with.
DBNull :(
If you've already decided to punish yourself by choosing Visual Basic for your project, then you're likely to be in the perfect headspace to use Microsoft Access as your database.
.NET uses a System.DBNull.Value
to represent a null (empty) value within the database. You may expect this to be equivalent to Nothing
in Visual Basic, but they are not quite the same. Nothing
in Visual Basic is a big source of confusion. It's not really a null
value, it's more equivalent to C#'s default
. It's a special token that will evaluate to the zero-value for each data type, 0
for Integer
, False
for Boolean
and Date.MinDate
for Date
. This is made even more confusing as the Visual Basic runtime treats a String
slightly differently and (usually) evaluates Nothing
to ""
. The exception is with .NET Framework, where it will remain as a Nothing
value.
To make things more confusing, as Visual Basic stands in the shadow of C#, reference to a proper notion of a null
value can be found scattered across the shared standard library, for example String.IsNullOrWhitespace
, even though the concept of null
does not exist.
' A .NET Framework example
Dim i1 As Integer = 0
Dim i2 As Integer = Nothing
Console.WriteLine(i1 Is Nothing) ' (False) 0 and Nothing are not strictly the same thing...
Console.WriteLine(Nothing = 0) ' (True) ...but they are equivalent
Console.WriteLine(i2 Is Nothing) ' (False) i2 has been evaluated to 0
Dim d1 As Date = Date.MinValue
Dim d2 As Date = Nothing
Console.WriteLine(d1 Is Nothing) ' (False) Date.MinValue and Nothing are not strictly the same thing...
Console.WriteLine(Nothing = Date.MinValue) ' (True) ...but they are equivalent
Console.WriteLine(d2 Is Nothing) ' (False) d2 has been evaluated to Date.MinValue
Dim s1 As String = ""
Dim s2 As String = Nothing
Console.WriteLine(s1 Is Nothing) ' (False) "" and Nothing are not strictly the same thing...
Console.WriteLine(Nothing = "") ' (True) ...but they are equivalent
Console.WriteLine(s2 Is Nothing) ' (True) s2 holds a real Nothing value... because .NET Framework
All this means that when using .NET Framework, since a string variable has the potential to retain Nothing
or ""
as discrete values, but the equality operator views them as equivalent, the following code will actually change the value held by myString
. Very confusing.
Dim myString As String = Nothing
If myString = "" Then
myString = ""
End If
This does is create a table of arbitrary rules you need to remember. You spend more time stressing over whether there are any gaps in your code than actually being productive. It's in the same direction as the lottery of results JavaScript can provide when using ==
.
Back to DBNull
. If we set name = NULL
in our SQL, we insert a null value that will be represented as DBNull
when we read it back into our program. The DBNull
value is required in order to bridge the gap between the .NET typing system and the database. As shown earlier, setting a Date
to Nothing
in Visual Basic will evaluate to Date.MinValue
, whereas a timestamp field in your database will probably allow a truly NULL
value.
If we wanted to find all users without a middle name we can run
SELECT * FROM users WHERE middlename IS NULL;
Seems simple enough? Wrong! It turns out a member of our team has opened the database in Access and manually deleted some incorrect middle names. A fairly innocent thing to do. But now our field could contain an empty string (""
) and there's no way of knowing from within Access.
So when working with a string field in our program we have three null values to worry about: ""
, Nothing
and DBNull
. This is fantastic news.
Imagine we fetch a user from our database, and we want to later check whether they have a middle name within our program.
Dim sql As String = "SELECT middlename FROM users;"
Dim cmd As New OleDBCommad(sql, connection)
connection.Open()
Dim reader As OleDBDataReader = cmd.ExecuteReader()
While reader.Read()
Dim middlename As String = reader.GetString(0)
If IsDBNull(middlename) Or middlename = "" Then
' Found a blank middlename
End If
End While
reader.Close()
connection.Close()
This all seems fine doesn't it? Ah... no, I'm afraid not.
error BC30311: Value of type 'DBNull' cannot be converted to 'String'.
We're not checking for null values when we blindly read each value into the middlename
string variable. Even though any potential null middlename values within our database sit within a string field, they cannot be interpreted as a string within Visual Basic, they are a rigid DBNull
value that we must check for. With all the crazy coercions that Visual Basic performs, you may have expected a DBNull
value to be evaluated as Nothing
when read into a string variable, but then you would have know way of knowing if your database happens to hold the real minimum date value or a NULL. A DBNull
value cannot fit within a slot designed for strings no matter how hard we try. DBNull
and Nothing
are two distinct values that .NET wants to keep separate.
When we call reader.GetString(0)
we are trying to interpret a DBNull
value found at index 0 as a string which crashes our program. If our value happened to be an empty string it would have been fine. Instead, we need to use the GetValue(0)
to retrieve the middlename successfully whether it's a DBNull
or a string. Since Visual Basic does not support union types, the Object
type is now the common denominator. We need to replace our middle name read with Dim middlename As Object = reader.GetValue(0)
.
...
While reader.Read()
Dim middlename As Object = reader.GetValue(0)
If IsDBNull(middlename) Or middlename = "" Then
' Found a blank middlename
End If
End While
...
Ok, this should be working now... But when we run our code, we get the same error. It turns out that, in order to evaluate middlename = ""
, Visual Basic tries to implicitly convert our DBNull
value into a string to see if they match. And as we already know, DBNull
cannot be coerced into a String.
...
While reader.Read()
Dim middlename As Object = reader.GetValue(0)
If IsDBNull(middlename) Then
' Found a blank middle name
ElseIf middlename = "" Then
' Found a blank middle name
End If
End While
...
Finally... we have some imperfect but working code. This is more a consequence of how we would expect the Or
operator to work, which leads us straight into the next issue.
And...?
Say we now want to make a list of every middle name we have in our database.
Dim sql As String = $"SELECT * FROM users;"
Dim cmd As New OleDbCommand(sql, connection)
connection.Open()
Dim adapter As New OleDbDataAdapter(cmd)
Dim ds As New DataSet()
adapter.Fill(ds, "users")
Dim users As New DataView(ds.Tables("users"), "", "", DataViewRowState.CurrentRows)
Dim middlenames As New HashSet(Of String)
For i As Integer = 0 To users.Count - 1
Dim middlename As Object = users.Item(i)("middlename")
If Not IsDBNull(middlename) And middlename <> "" Then
middlenames.Add(middlename) ' Store every non-blank middlename
End If
Next
connection.Close()
But we're still getting the same error as before...
error BC30311: Value of type 'DBNull' cannot be converted to 'String'.
Take a moment to think why this might be.
At some point our code is trying to convert a DBNull
value into a string. But how can this be? By the time we check for an empty string, we've already guarded against any DBNull
values? Well it turns out Visual Basic uses And
for logical AND, meaning every expression in the condition is evaluated to true or false in order to then apply a logical AND operation, comparing each bit like-for-like. There is no short-circuiting like you would expect in other languages if the first expression is False
.
Not only is this an easy cause of logical errors like this, but with dealing with heavy operands it can quickly create very inefficient unless you know what you're doing.
We could guard the empty string comparison that is causing the error by moving it into a separate `ElseIf` statement.
Dim middlename As Object = users.Item(i)("middlename")
If Not IsDBNull(middlename) Then
middlenames.Add(middlename)
ElseIf middlename <> "" Then
middlenames.Add(middlename)
End If
But what we are actually looking for is AndAlso
.
Dim middlename As Object = users.Item(i)("middlename")
If Not IsDBNull(middlename) AndAlso middlename <> "" Then
middlenames.Add(middlename)
End If
This can be a huge source of issues, which is exacerbated by the fact this is not how the average non-VB developer would expect these operators to work. Can you spot the problem with the function below?
Private Function UserProcessCount(user As String, processName As String) As Integer
Dim count As Integer = 0
Dim processes As Process() = Process.GetProcessesByName(currentProcess.ProcessName)
If processes.Length > 1 Then
For Each process As Process In processes
If process.ProcessName = processName And user = GetProcessUser(process) Then
count += 1
End If
Next
End If
Return count
End Function
The GetProcessUser()
and function will run for every single iteration over the processes running on the machine, and I wouldn't expect this to run instantly. This is a case where a missing AndAlso
stands in the way of potentially significant performance gains.
Or
works in a similar way with OrElse
, but incorrect use is less likely to occur and catch you out.
When languages opt for the plain english tokens and
, or
as alternatives to &&
and ||
, it often aids readability for those who don't read code for 6 hours a day. But Visual Basic has come full-circle back to the realm of confusing. And
and Or
tokens are great, but AndAlso
isn't even correct use of English. Any editor would note the "Also" is redundant, so why is it different here? If someone with no prior knowledge of Visual Basic syntax were shown And
and AndAlso
, I would expect they couldn't guess their semantic difference.
Just another unfortunate design decision that has stuck around from earlier versions of Visual Basic. Although VB.NET is marketed as a decent choice for beginners to develop software, and while it's still essentially a simplied version of C#, it is filled with many footguns that are ready to catch you out. While these common mistakes aren't quite as severe as undefined behaviour, frustratingly your VB.NET code will often semi-work and give you a false sense of security, making these bugs very difficult to spot.